Js Run for Lopp Again When It Is Complete Js Run for Loop Again When It Is Complete
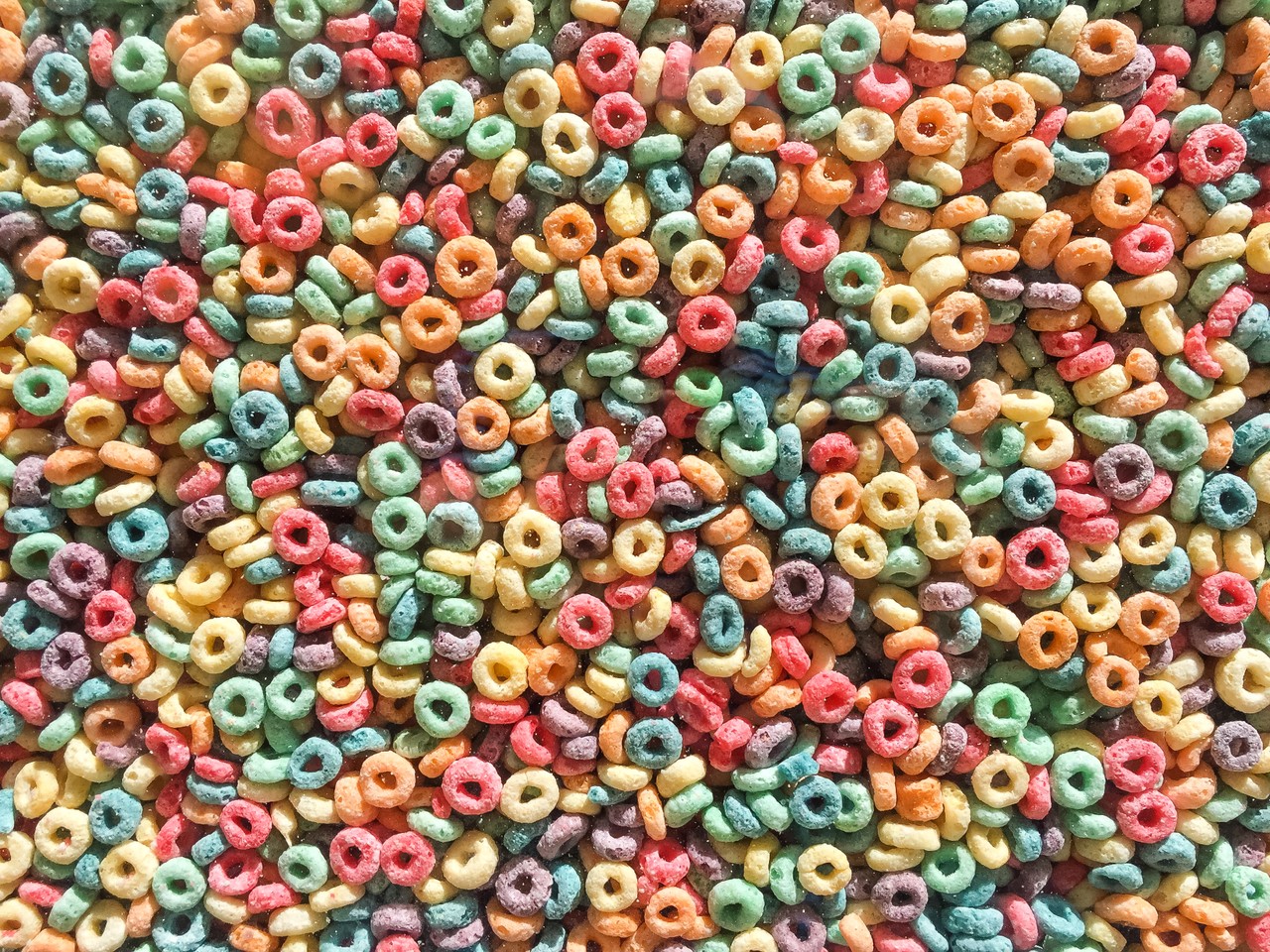
Loops are used in JavaScript to perform repeated tasks based on a condition. Conditions typically return true
or false
. A loop volition continue running until the divers condition returns faux
.
for
Loop
Syntax
for (initialization; status; finalExpression) { // lawmaking }
The for
loop consists of 3 optional expressions, followed by a code block:
-
initialization
- This expression runs earlier the execution of the offset loop, and is usually used to create a counter. -
condition
- This expression is checked each time earlier the loop runs. If it evaluates totrue
, theargument
or code in the loop is executed. If information technology evaluates toimitation
, the loop stops. And if this expression is omitted, it automatically evaluates totrue
. -
finalExpression
- This expression is executed afterwards each iteration of the loop. This is usually used to increment a counter, but tin exist used to decrement a counter instead.
Any of these three expressions or the the code in the lawmaking block tin can be omitted.
for
loops are commonly used to run code a gear up number of times. As well, y'all can utilize break
to leave the loop early, before the condition
expression evaluates to imitation
.
Examples
1. Iterate through integers from 0-8:
for (allow i = 0; i < 9; i++) { console.log(i); } // Output: // 0 // 1 // 2 // 3 // 4 // 5 // 6 // 7 // 8
2. Use break
to exit out of a for
loop earlier condition
is imitation
:
for (let i = 1; i < 10; i += two) { if (i === 7) { suspension; } console.log('Full elephants: ' + i); } // Output: // Total elephants: ane // Total elephants: 3 // Full elephants: v
Common Pitfall: Exceeding the Bounds of an Array
When iterating over an array, it'due south easy to accidentally exceed the bounds of the array.
For instance, your loop may endeavor to reference the 4th chemical element of an array with just 3 elements:
const arr = [ ane, 2, 3 ]; for (let i = 0; i <= arr.length; i++) { console.log(arr[i]); } // Output: // ane // 2 // 3 // undefined
At that place are two ways to fix this code: prepare condition
to either i < arr.length
or i <= arr.length - 1
.
for...in
Loop
Syntax
for (holding in object) { // code }
The for...in
loop iterates over the properties of an object. For each belongings, the lawmaking in the code block is executed.
Examples
one. Iterate over the backdrop of an object and log its proper noun and value to the console:
const capitals = { a: "Athens", b: "Belgrade", c: "Cairo" }; for (let primal in capitals) { console.log(fundamental + ": " + capitals[central]); } // Output: // a: Athens // b: Belgrade // c: Cairo
Mutual Pitfall: Unexpected Behavior When Iterating Over an Assortment
Though you can apply a for...in
loop to iterate over an assortment, it's recommended to use a regular for
or for...of
loop instead.
The for...in
loop can iterate over arrays and array-like objects, but it may non always access array indexes in gild.
Besides, the for...in
loop returns all properties and inherited properties for an array or array-similar object, which can lead to unexpected beliefs.
For example, this elementary loop works as expected:
const assortment = [1, 2, 3]; for (const i in array) { console.log(i); } // 0 // 1 // 2
But if something like a JS library you lot're using modifies the Array
paradigm directly, a for...in
loop will iterate over that, as well:
const array = [one, ii, 3]; Array.image.someMethod = true; for (const i in array) { console.log(i); } // 0 // i // ii // someMethod
Though modifying read-only prototypes like Array
or Object
direct goes confronting best practices, it could be an issue with some libraries or codebases.
Also, since the for...in
is meant for objects, it's much slower with arrays than other loops.
In short, just remember to but utilize for...in
loops to iterate over objects, not arrays.
for...of
Loop
Syntax
for (variable of object) { // lawmaking }
The for...of
loop iterates over the values of many types of iterables, including arrays, and special collection types like Set
and Map
. For each value in the iterable object, the lawmaking in the code block is executed.
Examples
1. Iterate over an array:
const arr = [ "Fred", "Tom", "Bob" ]; for (let i of arr) { panel.log(i); } // Output: // Fred // Tom // Bob
2. Iterate over a Map
:
const thou = new Map(); m.set(1, "black"); m.set(two, "blood-red"); for (let north of thousand) { console.log(northward); } // Output: // [1, black] // [2, ruby]
3. Iterate over a Set up
:
const south = new Set(); due south.add(1); s.add together("red"); for (let due north of south) { console.log(n); } // Output: // ane // scarlet
while
Loop
Syntax
while (condition) { // argument }
The while
loop starts by evaluating condition
. If condition
evaluates to true
, the code in the code block gets executed. If condition
evaluates to simulated
, the code in the code cake is not executed and the loop ends.
Examples:
- While a variable is less than 10, log it to the panel and increase it by 1:
let i = 1; while (i < 10) { console.log(i); i++; } // Output: // ane // two // 3 // 4 // 5 // 6 // 7 // 8 // nine
do...while
loop
Syntax:
practise { // statement } while (condition);
The do...while
loop is closely related to while
loop. In a do...while
loop, condition
is checked at the end of each iteration of the loop, rather than at the beginning before the loop runs.
This means that lawmaking in a exercise...while
loop is guaranteed to run at least one time, even if the condition
expression already evaluates to true
.
Instance:
- While a variable is less than 10, log it to the console and increment it past 1:
permit i = 1; practise { panel.log(i); i++; } while (i < x); // Output: // ane // 2 // 3 // 4 // 5 // 6 // 7 // 8 // 9
two. Push to an array, even if condition
evaluates to truthful
:
const myArray = []; permit i = 10; do { myArray.push(i); i++; } while (i < 10); console.log(myArray); // Output: // [10]
Learn to code for free. freeCodeCamp's open source curriculum has helped more than than 40,000 people become jobs as developers. Get started
Source: https://www.freecodecamp.org/news/javascript-loops-explained-for-loop-for/
0 Response to "Js Run for Lopp Again When It Is Complete Js Run for Loop Again When It Is Complete"
Post a Comment